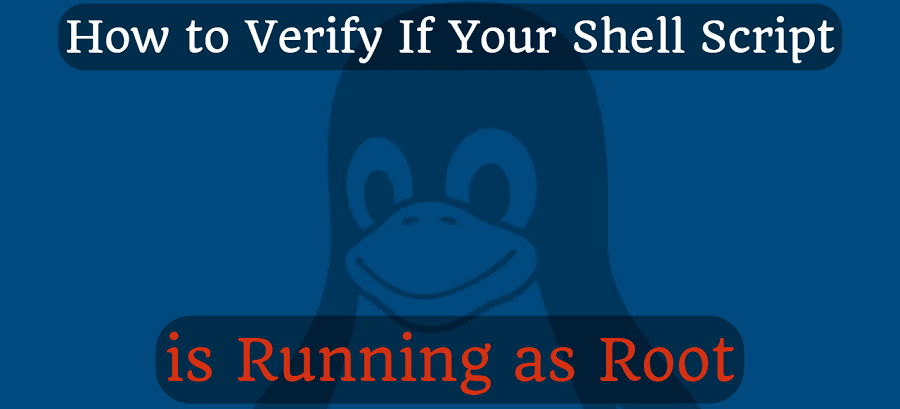
How to Verify If Your Shell Script is Running as Root
Often it is necessary to check if your shell script is running with root or not. The following code can be used to prevent others than the root user from running the script, even if they have file system level access. This will prevent unhandled errors in the code.
The code is simple:
[[ $EUID > 0 ]] && echo "You should run the script with root" && exit
It works very simply. The root user EUID is 0. If the EUID is greater than 0, it prints the text after it and then exits. In all other cases it continues to run.
If you want to use it in multiple scripts, it's a good idea to put it in a central file and then refer to it as a function, like this:
# Verify that the script is run by root
RootCheck() {
[[ $EUID > 0 ]] && echo "You should run the script with root" && exit
}
In this case, don't forget to call the external file in all your scripts:
. /path/to/central.file
That's all.
If you have any questions, feel free to ask in the comment section!
If you found this article useful and would like to show your appreciation, please consider making a small donation via PayPal. Your support will allow me to continue creating valuable content and make my blog even better. Thank you for your contribution!
Comments